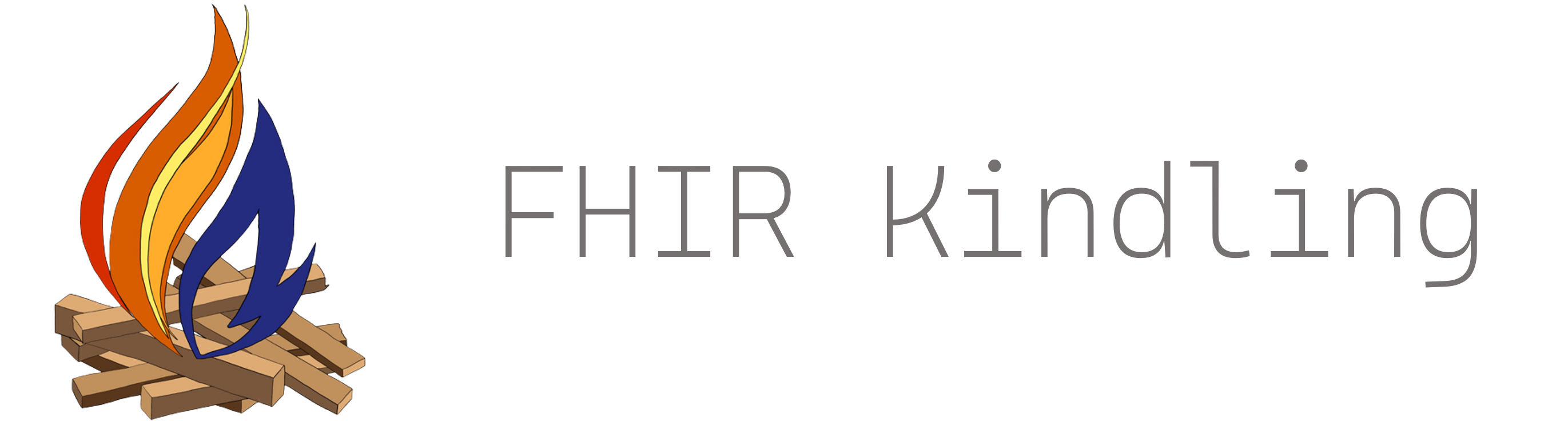
Python library for interacting with HL7 FHIR servers and resources. Resource validation and parsing powered by
pydantic and the fhir.resources library.
Provides a simple interface for synchronous and asynchronous CRUD operations for resources and bundles,
as well as resource transfer between servers.
Datascience features include flattening of resources and bundles into tabular format (pandas dataframe) and plotting
methods for resources and bundles can optionally be included with the ds
extra.
Warning
Under construction. This documentation is not complete.
Features
- Connect to FHIR (Version R4) servers using different auth methods
- Sync/async CRUD operations for bundles and resource
- Resource transfer between servers
- Resource/Bundle serialization to CSV
- Resource generation for synthetic data sets
Installation
To install the package via pypi without any extra dependencies, use the following command:
pip install fhir_kindling
poetry add fhir_kindling
Extras (optional)
Fhir kindling can be used with the following extras:
Data science
Install the package with the ds
extra to get the following features:
flatten
method for flattening a resource into a tabular format (pandas dataframe)flatten_bundle
method for flattening a bundle into a tabular format (pandas dataframe)- Plotly based plotting methods for resources and bundles
pip install fhir_kindling[ds]
Quick start
Connecting to a FHIR server
from fhir_kindling import FhirServer
# Connect with basic auth
basic_auth_server = FhirServer("https://fhir.server/fhir", username="admin", password="admin")
# Connect with static token
token_server = FhirServer("https://fhir.server/fhir", token="your_token")
# Connect using oauth2/oidc
oidc_server = FhirServer("https://fhir.server/fhir", client_id="client_id", client_secret="secret",
oidc_provider_url="url")
# Print the server's capability statement
print(basic_auth_server.capabilities)
Query resources from the server
from fhir_kindling import FhirServer
from fhir.resources.patient import Patient
# Connect using oauth2/oidc
oidc_server = FhirServer("https://fhir.server/fhir", client_id="client_id", client_secret="secret",
oidc_provider_url="url")
# query all patients on the server
query = oidc_server.query(Patient, output_format="json").all()
print(query.response)
# Query resources based on name of resource
query = oidc_server.query("Patient", output_format="json").all()
print(query.response)
Credits
All the FHIR resource validation is done via the fhir.resources package.